파이썬으로 3D 게임 만들기
필요 라이브러리: Ursina
Ursina 설치 방법
If you are a Windows user:
1) Open CMD or Powershell
2) Type "pip install ursina"
If you are a PyCharm user:
1) Type "ursina import *"
2) Click on "Install uninstalled libraries"
# 기초
기초 - 1. 자동 무한회전하는 육각면체 만들기
1
2
3
4
5
6
7
8
9
10
11
|
from ursina import *
app = Ursina()
cube = Entity(model='cube', color=color.red, texture='white_cube', scale=2)
def update():
cube.rotation_x = cube.rotation_x + 0.25
cube.rotation_y = cube.rotation_y + 0.5
app.run()
|
cs |
위 코드를 실행하면 아래와 같은 육각면체가 무한회전하는 것을 확인할 수 있다.
색깔과 각도를 조금씩 바꿔서 해보자!
1
2
3
4
5
6
7
8
9
10
11
|
from ursina import *
app = Ursina()
cube = Entity(model='cube', color=color.green, texture='white_cube', scale=2)
def update():
cube.rotation_x = cube.rotation_x + 0.5
cube.rotation_y = cube.rotation_y + 0.15
app.run()
|
cs |
기초 - 2. 방향키로 육각면체 이동/회전하기
자동으로 움직이는 3D 도형이 아닌 방향키를 이용해서 3D 도형을 이동시켜 보자.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
from ursina import *
app = Ursina()
player = Entity(model='cube', color=color.blue, scale_y=2)
def update():
player.x += held_keys["d"] * 0.1
player.x -= held_keys["a"] * 0.1
player.y += held_keys["w"] * 0.1
player.y -= held_keys["s"] * 0.1
player.rotation_x += held_keys["r"] * 5
player.rotation_y += held_keys["r"] * 5
app.run()
|
cs |
W 상
A 좌
D 우
S 하
R 회전
방향키를 만들었습니다!
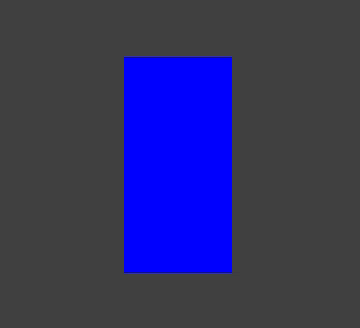
기초 - 3. 초기 맵 만들어보기
맵을 만들어서 스페이스바를 누르면 점프하도록 만들어봤습니다. 방향키 역시 그대로 WASD 키를 이용했습니다.
중급 - 1. Tic Tac Toe 게임 만들기!
출처: https://github.com/pokepetter/ursina/blob/master/samples/tic_tac_toe.py
틱택토 게임은 사실 3D 게임이 아닌 2D 게임입니다. 그렇지만, Ursina 엔진의 player와 마우스 이동, 클릭 시 상태 변환에 대한 이해를 기를 수 있는 간단한 프로젝트입니다.
게임 화면
아래 코드를 그대로 입력하면, 그리고 Ursina library를 제대로 설치만 했다면 다른 외부 파일 없이 잘 작동할 것입니다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
|
from ursina import *
if __name__ == '__main__':
app = Ursina()
camera.orthographic = True
camera.fov = 4
camera.position = (1, 1)
Text.default_resolution *= 2
player = Entity(name='o', color=color.azure)
cursor = Tooltip(player.name, color=player.color, origin=(0,0), scale=4, enabled=True)
cursor.background.color = color.clear
bg = Entity(parent=scene, model='quad', texture='shore', scale=(16,8), z=10, color=color.light_gray)
mouse.visible = False
# create a matrix to store the buttons in. makes it easier to check for victory
board = [[None for x in range(3)] for y in range(3)]
for y in range(3):
for x in range(3):
b = Button(parent=scene, position=(x,y))
board[x][y] = b
def on_click(b=b):
b.text = player.name
b.color = player.color
b.collision = False
check_for_victory()
if player.name == 'o':
player.name = 'x'
player.color = color.orange
else:
player.name = 'o'
player.color = color.azure
cursor.text = player.name
cursor.color = player.color
b.on_click = on_click
def check_for_victory():
name = player.name
won = (
(board[0][0].text == name and board[1][0].text == name and board[2][0].text == name) or # across the bottom
(board[0][1].text == name and board[1][1].text == name and board[2][1].text == name) or # across the middle
(board[0][2].text == name and board[1][2].text == name and board[2][2].text == name) or # across the top
(board[0][0].text == name and board[0][1].text == name and board[0][2].text == name) or # down the left side
(board[1][0].text == name and board[1][1].text == name and board[1][2].text == name) or # down the middle
(board[2][0].text == name and board[2][1].text == name and board[2][2].text == name) or # down the right side
(board[0][0].text == name and board[1][1].text == name and board[2][2].text == name) or # diagonal /
(board[0][2].text == name and board[1][1].text == name and board[2][0].text == name)) # diagonal \
if won:
print('winner is:', name)
destroy(cursor)
mouse.visible = True
Panel(z=1, scale=10, model='quad')
t = Text(f'player\n{name}\nwon!', scale=3, origin=(0,0), background=True)
t.create_background(padding=(.5,.25), radius=Text.size/2)
t.background.color = player.color.tint(-.2)
if __name__ == '__main__':
app.run()
|
cs |
중급 - 2. 게임 인벤토리 창 만들기!
게임 화면
RPG 게임을 하면 아이템 인벤토리 창을 확인해야 할 때가 있죠. 그런 인벤토리 창도 Ursina 엔진을 이용해서 제작할 수 있습니다. 웬만한 게임 로직은 Ursina 안에서 전부 해결 가능한 것 같습니다.
중급 - 3. 슈퍼마리오-like 게임
게임 화면
아주 간단한 게임이죠. 자신의 캐릭터를 목표지점까지 이동시키면 미션이 완료되는 게임입니다.
이상 Python Ursina에 대해 알아봤습니다. 재미난 게임을 여럿 만들 수 있다는 점이 흥미로웠고, 라이브러리 설치 한 번만 하면 다양하고 유용한 기능을 많이 사용할 수 있다는 점이 흥미로웠습니다.
위 기본 프로젝트를 토대로 그래픽 이미지와 스토리만 추가하면 더 재미나고 흥미로운 게임을 제작할 수 있을 것 같네요.
'공부 > 파이썬 Python' 카테고리의 다른 글
비밀번호 강력도 확인 (Password Strength Checker) with Python (0) | 2022.01.26 |
---|---|
Pomodoro 뽀모도로 공부 타이머 만들기 (feat. Python, Threading) (0) | 2022.01.23 |
Python 3D 플로팅 연습하기 (0) | 2022.01.04 |
Python에서 나만의 HTTP Server 만들기 (0) | 2022.01.03 |
Matplotlib 애니메이션으로 Linear Regression 표현하기 (0) | 2021.12.26 |
댓글